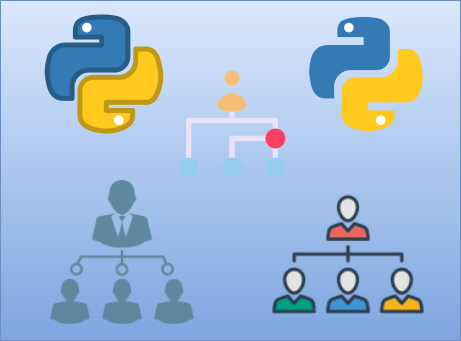
In this tutorial we will learn how to create a python class and go through several python class examples while explaining different concepts in classes.
Table Of Contents
- Why do we need Classes
- Most Basic Python Class Example
- Python Class example with a constructor
- Python Class example on methods
- Python Class Example on Inheritance.
- Conclusion.
Prerequisites
Before diving in be sure to have at least a basic understanding of Object-Oriented Programming in Python3.
What are classes and why do we need them anyway?
You might be asking yourself why we use classes anyway. A class is used to bundle together data and functionality into one entity making it possible to reuse code and encapsulation.
Using classes helps you organize your code by allowing you to group data and functionality into specific classes instead of having them scattered in your code.
classes make it possible to define all your data and functionality in one class which we refer to as the parent class and have many other child classes inheriting all the data and functionality of parent class. The inheriting class or the child class can override some of the characteristics of the parent class. This concept is referred to as inheritance.
A class acts as a blueprint for creating objects(instances). It is good to note that Everything in python is an object. Even a class itself is an object of type class.
The Most Basic Python class example
The following is the most basic and simplest class you can create in python.
class Car:
pass
This is a class that does nothing. The pass keyword simple means do not do anything or execute nothing.
Python Class Example with a constructor.
A constructor in classes is a special method that is executed or called immediately when a class is instantiated. A constructor method does not need to be called for it to execute. A constructor function in python will always have the name __init__ . Be sure to always have double underscores before the name init and double underscores after.
class Car:
def __init__(self, model, year):
self.model = model
self.year = year
Looking at the python class example above we have introduced something new, the self keyword. It is used to represent or to refer to the specific and current instance of the class. It helps in accessing the methods and attributes of a class.
Python Class Example With Methods
A method is a function within a class that operates on or manipulates the class data. Methods can further be classified according to their purpose in the class. Some of these classifications include.
- Instance methods: They are just like normal functions, they must always have the parameter self. They are mainly used in cases where there is need to access and modify properties of an object. They do not need any decorator.
- Class methods: These are methods that can be accessed directly through the class. The special attribute of class methods is that they do not require an object to be created. They take an attribute called cls(means class) by convention but can be changed to a different name. To define this kind of method we use the a decorator called @classmethod.
Class methods are used in the following scenarios:
-Where there is need to access the state of the class.
-Where there is need to make changes on all instances of a
particular class.
- Static methods: Loosely speaking, static methods are independent plain functions found in classes. They do not need either self or cls as special parameters. They are used in cases where a function does not need any class or object relation but will still be used to achieve a given functionality within the class. It is important to understand that a static function cannot access or modify the class or instance variables. They use the @static decorator.
We will use the following python class example to see the actual differences in code.
class Person:
def __init__(self, fname, lname, gender):
self.fname = fname
self.lname = lname
self.gender = gender
def print_details(self):
"""Example of an instance method"""
print("Hey, I am an instance method")
print(f"{self.fname} {self.lname} {self.gender}")
@classmethod
def get_loan(cls, loan):
"""Example of an instance method"""
print("Hey, I am a class method")
print(f"You requested for a ${loan} loan")
@staticmethod
def can_vote(age):
"""Example of an instance method"""
print("Hey, I am a static method")
if age >= 18:
print("Can vote")
else:
print("Cannot vote")
# To call a static method you must first create an instance.
person1 = Person("John", "Doe", "male")
person1.print_details()
# To call a classmethod or a static method you do not necessarily need an instance.
Person.can_vote(20)
Person.get_loan(1000)
Output
Hey, I am an instance method
John Doe male
Hey, I am a static method
Can vote
Hey, I am a class method
You requested for a $1000 loan
Python class example showing inheritance
To demonstrate inheritance we are going to consider a parent class called Person.
Python class example showing the parent class
class Person:
def __init__(self, firstname, lastname, gender):
self.firstname = firstname
self.lastname = lastname
self.gender = gender
def print_details(self):
print(f"{self.firstname} {self.lastname} {self.gender}")
The above example shows a Person class with the init constructor method taking firstname, lastname and gender whenever the class is instantiated. This class contains one method called print_details that prints the details of the class when called.creating the
Python class example showing the child class
A child class in python is a class that inherits from at least one parent. Creating a child class is very simple, just give the parent class as a parameter to the child class when creating the child class.
class Person:
def __init__(self, firstname, lastname, gender):
self.firstname = firstname
self.lastname = lastname
self.gender = gender
def print_details(self):
print(f"{self.firstname} {self.lastname} {self.gender}")
class Teacher(Person):
pass
We created a child class called teacher and to make sure it inherits from the Person class (because a teacher is a person) we passed the Person class as an argument to the Teacher class. We used the pass keyword since our class does nothing, the pass keyword tells python that this class does not do anything but note that it still has all the attributes and methods from the parent class.
Python class example showing how to create a child class instance
The process of creating a class instance is referred to as instantiation. We will create an instance of the python class example shown above. Our full program will now look like this.
class Person:
def __init__(self, firstname, lastname, gender):
self.firstname = firstname
self.lastname = lastname
self.gender = gender
def print_details(self):
print(f"{self.firstname} {self.lastname} {self.gender}")
class Teacher(Person):
pass
teacher1 = Teacher("John", "Doe", "Male")
teacher1.print_details()
In the last section of our program, we created an instance of the teacher class and since the teacher class inherits from the Person Class we had to give all the arguments required by the init method of the parent class. Notice that the arguments in the __init__ method will always be given as arguments when creating an instance of the class.
CONCLUSION.
In this tutorial we went through different python class examples while explaining various concepts in the classes.
For a deeper dive into classes I recommend you to go through the official documentation for classes.